Simplest Blazor IOT Dashboard
Recently I was learning Blazor, here is the first taste.
Demo :
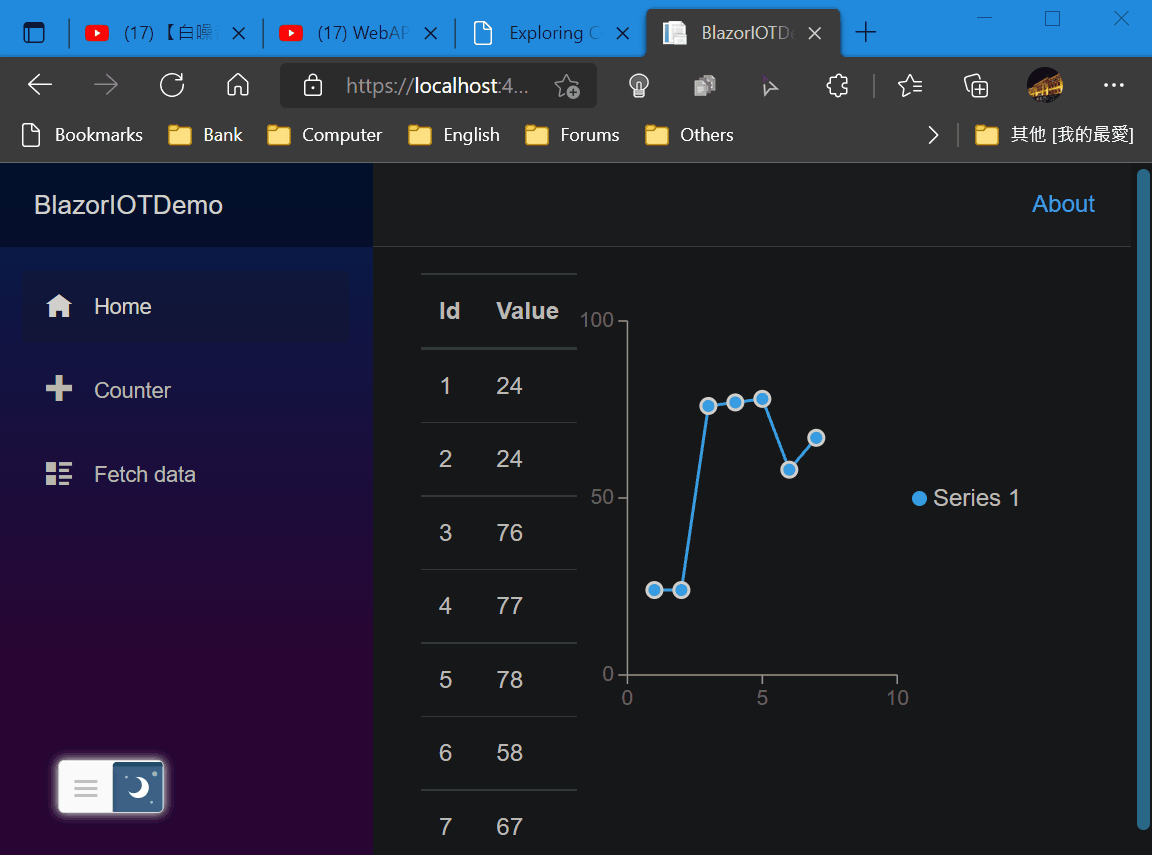
Step
- Create a “Blazor Server Application” project.
- Named it “BlazorIOTDemo” or whatever you like.
- Add “MyDataDto.cs” in Data folder.
public class MyDataDto { public int Id { get; set; } public int Value { get; set; } }
- Add “DataService.cs” in Data folder.
public class DataService { public DataService() { this._timer = new Timer(UpdateValues, null, this._updateInterval, this._updateInterval); } public event EventHandler<List<MyDataDto>> DataChanged; private Timer _timer; private readonly TimeSpan _updateInterval = TimeSpan.FromMilliseconds(1000); private void UpdateValues(object state) { var myDataDtos = new List<MyDataDto> { new MyDataDto() { Id = 1, Value = new Random().Next(1, 80) }, new MyDataDto() { Id = 2, Value = new Random().Next(10, 80) }, new MyDataDto() { Id = 3, Value = new Random().Next(20, 80) }, new MyDataDto() { Id = 4, Value = new Random().Next(30, 80) }, new MyDataDto() { Id = 5, Value = new Random().Next(40, 80) }, new MyDataDto() { Id = 6, Value = new Random().Next(50, 80) }, new MyDataDto() { Id = 7, Value = new Random().Next(60, 80) } }; DataChanged?.Invoke(this, myDataDtos); } }
- Configure service in "Startup.cs"
It’ll be better to use interface.
services.AddSingleton<DataService>();
- Alter the content in “Index.razor” to this
Project structure: